Neo4j from JavaScript
Important - page not maintained
This page is no longer being maintained and its content may be out of date. For the latest guidance, please visit the Getting Started Manual . |
If you are a JavaScript developer, this guide provides an overview of options for connecting to Neo4j. While this guide is not comprehensive it will introduce the different Drivers and link to the relevant resources.
You should be familiar with graph database concepts and the property graph model. You should have created an Neo4j AuraDB cloud instance, or installed Neo4j locally
Intermediate
Neo4j for JavaScript Developers
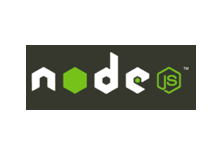
Neo4j provides drivers which allow you to make a connection to the database and develop applications which create, read, update, and delete information from the graph.
You can use the official driver for JavaScript (neo4j-driver) or connect via HTTP using the request
module or any of our community drivers.
Learn with GraphAcademy
Building Neo4j Applications with Node.js
In this free course, we walk through the steps to integrate Neo4j into your Node.js projects. You will learn about the Neo4j JavaScript Driver, how sessions and transactions work and how to query Neo4j from an existing application.
Building Neo4j Applications with TypeScript
Building Neo4j Applications with TypeScript course is a shorter course, with an estimated duration of two hours, aimed at TypeScript developers that are interested in learning the TypeScript specific features which add type-checking to any interactions you have with a Neo4j instance.
Neo4j Javascript Driver
The Neo4j JavaScript driver is officially supported by Neo4j and connects to the database using the binary protocol. It aims to be minimal, while being idiomatic to JavaScript, allowing you to subscribe to a stream of responses, errors and completion events.
npm install neo4j-driver
const neo4j = require('neo4j-driver')
const driver = neo4j.driver(uri, neo4j.auth.basic(user, password))
const session = driver.session()
const personName = 'Alice'
try {
const result = await session.run(
'CREATE (a:Person {name: $name}) RETURN a',
{ name: personName }
)
const singleRecord = result.records[0]
const node = singleRecord.get(0)
console.log(node.properties.name)
} finally {
await session.close()
}
// on application exit:
await driver.close()
Driver Configuration
From Neo4j version 4.0 and onwards, the default encryption setting is off by default and Neo4j will no longer generate self-signed certificates.
This applies to default installations, installations through Neo4j Desktop and Docker images.
You can verify the encryption level of your server by checking the dbms.connector.bolt.enabled
key in neo4j.conf
.
Certificate Type | Neo4j Causal Cluster | Neo4j Standalone Server | Direct Connection to Cluster Member |
---|---|---|---|
Unencrypted |
|
|
|
Encrypted with Full Certificate |
|
|
|
Encrypted with Self-Signed Certificate |
|
|
|
|
N/A |
N/A |
Please review your SSL Framework settings when going into production. If necessary, you can also generate certificates for Neo4j with Letsencrypt
Name |
Version |
Authors |
neo4j-driver |
5.8.0 |
The Neo4j Team |
The Example Project
The Neo4j example project is a small, one page webapp for the movies database built into the Neo4j tutorial. The front-end page is the same for all drivers: movie search, movie details, and a graph visualization of actors and movies. Each backend implementation shows you how to connect to Neo4j from each of the different languages and drivers.
You can learn more about our small, consistent example project across many different language drivers here. You will find the implementations for all drivers as individual GitHub repositories, which you can clone and deploy directly.
Neo4j Community Drivers
Members of the each programming language community have invested a lot of time and love to develop each one of the community drivers for Neo4j, so if you use any one of them, please provide feedback to the authors.
The community drivers have been graciously contributed by the Neo4j community. Many of them are fully featured and well-maintained, but some may not be. Neo4j does not take any responsibility for their usability. |
Using the HTTP-Endpoint directly
You can use something as simple as the request
node-module to send queries to and receive responses from Neo4j.
The endpoint protocol and formats are explained in detail in the Neo4j Manual.
It enables you do to much more, e.g. sending many statements per request or keeping transactions open across multiple requests.
Here is a very simple example:
var r=require("request");
var txUrl = "http://localhost:7474/db/neo4j/tx/commit"; // neo4j is the database name
function cypher(query,params,cb) {
r.post({uri:txUrl,
json:{statements:[{statement:query,parameters:params}]}},
function(err,res) { cb(err,res.body)})
}
var query="MATCH (n:User) RETURN n, labels(n) as l LIMIT {limit}"
var params={limit: 10}
var cb=function(err,data) { console.log(JSON.stringify(data)) }
cypher(query,params,cb)
{"results":[
{"columns":["n","l"],
"data":[
{"row":[{"name":"Aran"},["User"]]}
]
}],
"errors":[]}